JO Menu
A client side library to use the JO Menu in your scripts.
JO Menu is a NUI menu fully optimized and mouse and keyboard ready.
Introducing The Jump On Menu: Revolutionizing Menu Creation for Your RedM Script!
The Jump On Menu offers a new way to build menus for your RedM script, seamlessly blending with the authentic design of Red Dead Redemption II. This new menu system is significantly more flexible and user-friendly compared to the MenuAPI in your current framework. Actions are now directly linked to individual buttons instead of the global menu, simplifying both setup and usage.
Your players will love the innovative features, including the grid layout, color picker, and smooth menu animations. Enhance their gaming experience and rediscover the joy of development with The Jump On Menu.
Previews
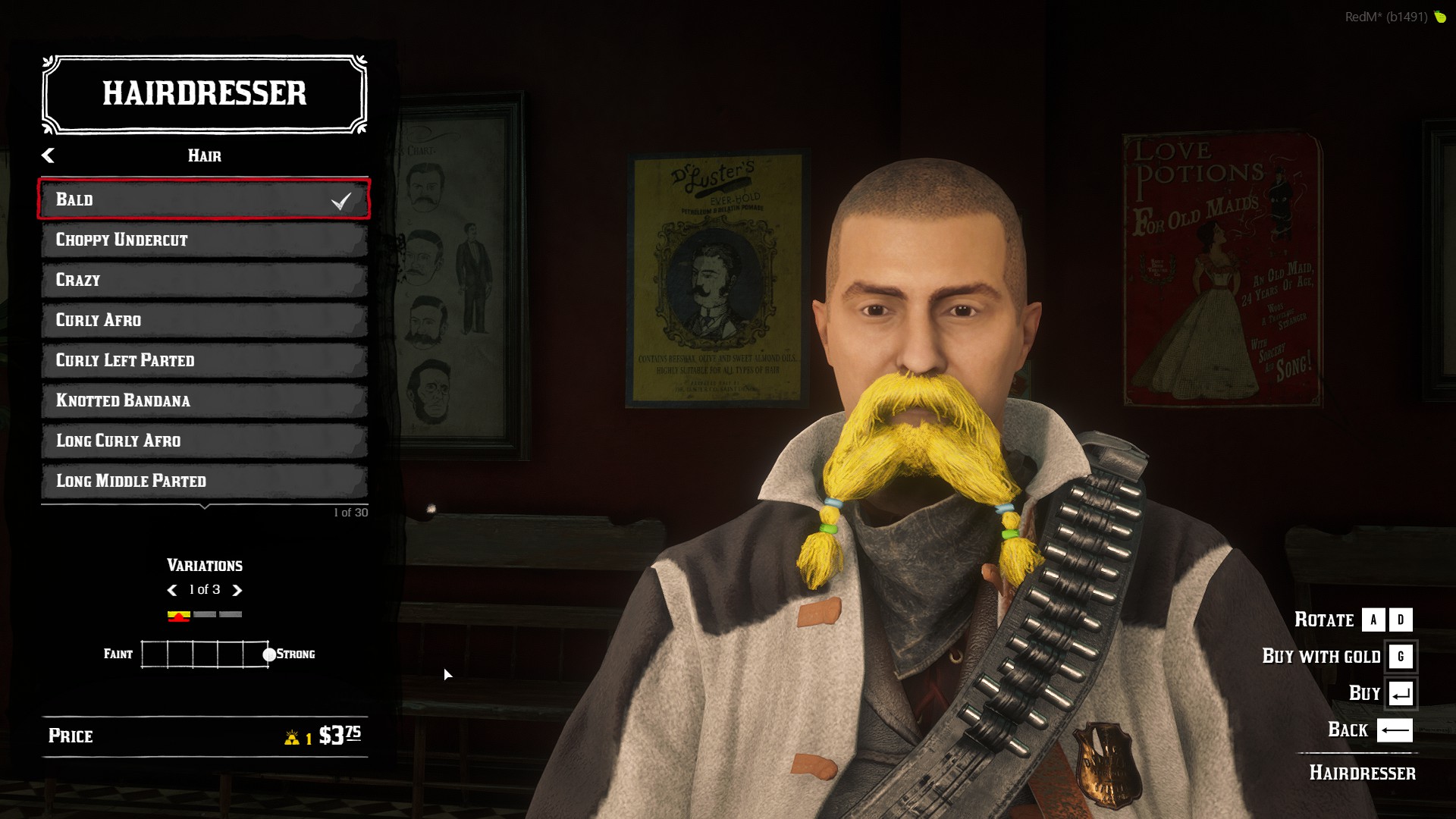
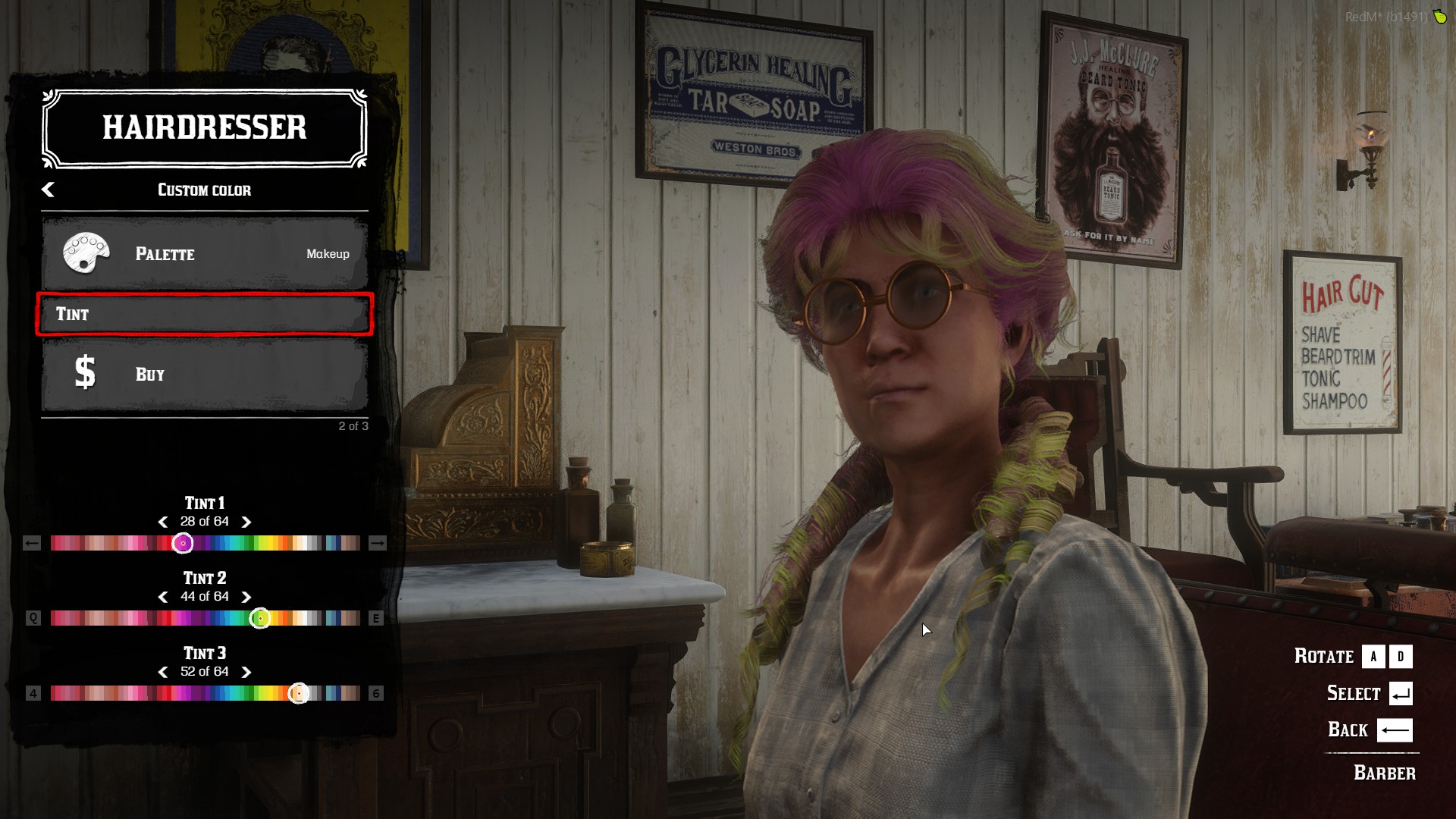
Usage
Download an example of menu resource
Constructor
Syntax
jo.menu.create(id,data)
Parameters
id
: string
The unique ID of the menu
data
: table
data.title
: string - The big title of the menudata.subtitle
: string - The subtitle of the menudata.numberOnScreen
: integer (default: 8) - The subtitle of the menu Optionaldata.onEnter
: function - Fired when the menu is opened Optionaldata.onBack
: function - Fired when the backspace/Escape is pressed Optionaldata.onExit
: function - Fired when the menu is exited Optional
Return value
Type: Menu
Return a Menu class
Example
--The unique ID of the menu
local id = 'UniqueId1'
--The menu data
local data = {
title = 'Menu', --The big title of the menu
subtitle = 'Elements', --The subtitle of the menu
numberOnScreen = 8, --The number of item display before add a scroll
onEnter = function(currentData)
print('Enter in menu '..id)
end,
onBack = function(currentData)
print('Backspace/Esc pressed in menu '..id)
end,
onExit = function(currentData)
print('Exit menu '..id)
end,
}
local menu = jo.menu.create(id,data)
Instance Methods
menu:addItem
A method to add an item to the menu
Syntax
menu:addItem({
definition
})
Keys for the item definition
title
: string
The item label
child
: string Optional
the menu to open when Enter is pressed
default: false
visible
: boolean (default: true) Optional
if the item is visible (hide in the menu)
default: true
data
: table Optional
variable to store datas in the item
description
: string Optional
the description
prefix
: string Optional
the little icon before the title from
nui\menu\assets\images\icons
folder
icon
: string Optional
the left icon filename from
nui\menu\assets\images\icons
folder
iconRight
: string Optional
the right icon filename from
nui\menu\assets\images\icons
folder
iconClass
: string Optional
CSS class for the icon
price
: table (default: false)Optional
the price of the item. Use O to display "free" default : false
price.money
: float - the price in $ Optionalprice.gold
: float - the price in gold Optional
priceTitle
: *string Optional
replace the "Price" label
priceRight
: boolean Optional
display the price at the right of the item title
statistics
: table Optional
the list of statistics
disabled
: boolean Optional
if the item is disabled (grey in the menu)
textRight
: string Optional
the label displayed at the right of the item
previewPalette
: boolean Optional
display a color square at the right of the item
default: true
sliders
: table
The list of sliders
onActive
: function
Fire when the item is enter
onClick
: function
Fire when Enter is pressed
onChange
: function
Fire when a slider value change
onExit
: function
Fire when the item is exit
Example
local menu = jo.menu.create('menu',{})
menu:addItem({
title = "Item 1",
price = {money = 10.2},
onActive = function(currentData)
print('Item 1 active')
end,
onClick = function(currentData)
print('Clicked on item 1')
end,
onChange = function(currentData)
print('Something change in the item 1')
end,
onExit = function(currentData)
print('Exit the item 1')
end
})
menu:addItem({
title = "Item 2",
price = {money = 10.2},
onActive = function(currentData)
print('Item 2 active')
end,
onClick = function(currentData)
print('Clicked on item 2')
end,
onChange = function(currentData)
print('Something change in the item 2')
end,
onExit = function(currentData)
print('Exit the item 2')
end
})
menu:send()
jo.menu.setCurrentMenu('menu')
jo.menu.show(true)
menu:addItems
A method to add multiples items
Syntax
menu:addItems(items)
Parameters
items
: table
List of items (see item definition)
Example
local items = {
{title = "Item 1"},
{title = "Item 2"}
}
local menu = jo.menu.create('menu',{})
menu:addItems(items)
menu:send()
jo.menu.setCurrentMenu('menu')
jo.menu.show(true)
menu:refresh()
A method to send the new data to the NUI
Syntax
menu:refresh()
Example
local menu = jo.menu.create(id,data)
menu:addItem({title="Item 1"})
menu:send()
menu:addItem({title="Item 2"})
menu:refresh()
menu:reset()
A method to put back the cursor on the first item
Syntax
menu:reset()
Example
menu:reset()
menu:send()
A method to send the menu to the NUI
Syntax
menu:send()
Example
local menu = jo.menu.create(id,data)
menu:send()
Functions
jo.menu.setCurrentMenu()
A function to define a menu like the current menuu
Syntax
jo.menu.setCurrentMenu(id, keepHistoric, resetMenu)
Parameters
id
: string
The unique ID of the menu
keepHistoric
: boolean
If you want keep the menu historic to open the previous menu when backspace/esc is pressed
default: true
resetMenu
: boolean
If you want reset the cursor to the first item of the menu
default: true
Example
local id = "UniqueId1"
local keepHistoric = true
local resetMenu = true
jo.menu.setCurrentMenu(id, keepHistoric, resetMenu)
jo.menu.delete()
A function to delete a menu
Syntax
jo.menu.delete(id)
Parameters
id
: string
The unique ID of the menu
Example
local id = "UniqueId1"
jo.menu.delete(id)
jo.menu.isOpen()
A function to know if a menu is opened
Syntax
jo.menu.isOpen()
Return value
Type: boolean
Return
true
if a menu is opened
Example
local isOpen = jo.menu.isOpen()
print(isOpen)
jo.menu.show()
The function to show/toggle a menu
Syntax
jo.menu.show(visible, keepInput, hideRadar)
Parameters
visible
: boolean
If the menu is visible or not
keepInput
: boolean
If you want keep the control on the game
default: true
hideRadar
: boolean
If you want hide the radar
default: true
Example
local visible = true
local keepInput = true
local hideRadar = true
jo.menu.show(visible,keepInput,hideRadar)
Variables
CurrentData
The argument pass on each function
keys
CurrentData.menu
: string
The unique ID of the menu
CurrentData.item
: table
The item active in the menu
Replace your old menu
By default, the library is released with resource to replace the old framework menu.
VORP
Replace the vorp_menu
resource by the one delivered with the library
RSG
Replace the rsg-menubase
resource by the one delivered with the library
RedEM:RP
Replace the redemrp_menu_base
resource by the one delivered with the library
New assets
Add a new icon
Add you .png file in the nui\menu\assets\images\icons
folder
Item variations
Sliders
4 types of sliders are available on the menu: Default, Grid, Palette & Switch.
You can use multiples sliders on the same item.
Use currentData.item.sliders[X].value
to get the current value of the slider.
Here is an example of an item with sliders of the 4 types:
Default
The default slider based on the original game design. Usefull to choose between item variations like clothes.
Syntax
{title = "", current = 1, values = {item1,item2,..}}
Keys
title
: string
The label on the top of variations
current
: integer
The current item selected
values
: table
The table of item variations. 1 entry = 1 rectangle
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
sliders = {
{
title = 'Variations',
current = 2,
values = {
"value1",
{var = 4},
{yourKey = "your Value"},
'value2',
5,
10,
}
},
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Grid
The grid slider is usefull to define a value between a min and max value.
You can use one or two dimensions of slider.
One dimension: Two dimensions:
TIP
To get the values of the slider, .value
is a table with two arguments:currentData.item.sliders[X].value[1]
for the horizontal axe (or for one dimension slider)currentData.item.sliders[X].value[2]
for the vertical axe
Syntax
{type = "grid", labels = {'left','right','up','down'}, values = {
{current = 0.5,max = 1.0, min = -1.0},
{current = 0.5,max = 10.0, min = 0.0}, --for two dimensions
}}
Keys
type
: string
The type of slider
labels
: table
The 4 labels in the order : left, right, top, bottom
values
: table
The slider definitions.
1 entry = 1 dimension slider
2 entries = 2 dimensions slidersvalues[x].current
: float - the current value of the slidervalues[x].min
: float - the minimal value (cursor on the full left/top)values[x].max
: float - the minimal value (cursor on the full right/bottom)
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
sliders = {
{
type = "grid",
labels = {'left','right','up','down'},
values = {
{current = 0.5,max = 1.0, min = -1.0},
{current = 0.5,max = 10.0, min = 0.0}, --for two dimensions
}
},
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Palette
The palette slider is usefull to select a color.
Syntax
{type = "palette", title = "tint", tint = "tint_makeup", max = 63, current = 14}
Keys
type
: string
The type of slider
title
: table
The top label on the slider
tint
: string
The name of the gradient :
"tint_generic_clean", "tint_hair", "tint_horse", "tint_horse_leather", "tint_leather" & "tint_makeup"
max
: integer
The number of varations
current
: integer
The current variation
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
sliders = {
{type = "palette", title = "tint", tint = "tint_makeup", max = 63, current = 14}
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Switch
The switch slider is display on the right of the item label.
Syntax
{type = "switch", current = 1, values = {
{label = "", data = {}},
{label = "", data = {}}
}}
Keys
type
: string
The type of slider
current
: integer
The current variation
values
: table
The list of variations
values[x].label
is the label displayed
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
sliders = {
{ type = "switch", current = 1, values = {
{label = "value 1", myKey = 4},
{label = "value 2", data = "what you want"}
}
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Statistics
Statistics table is displayed at the bottom of the menu. 5 types of statistics are available: Bar, Bar-style, Icon, Texts and Weapon-bar
Multiple statistics can be use for the same item.
Here is an example of an item with 5 statistics of the 5 types:
Bar
A statistic with 10 bars
Syntax
{label = "", type = "bar", value = {current,max}}
Keys
label
: string
the left label
type
: string
value
: table
For the left to the right,
current
are white,max
are grey, all the rest is dark greyvalue.current
: integer (0<>10 - the number of white barvalue.max
: integer (0<>10 - the number of grey bar
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
statistics = {
{label = "The label", type = "bar", value = {3,8}}
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Bar-style
A statistic with unlimted bar defined with CSS classes
Syntax
{label = "", type = "bar-style", value = {'','',''}}
Keys
label
: string
the left label
type
: string
value
: table
A list of string to define the CSS classes of bar, 1 string = 1 bar
If the string is empty, the bar is dark grey. CSS classes:
active
: Opacity = 1fgold
: Goldfred
: Redpossible
: Opacity = 0.5CSS classes can be combinated
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
statistics = {
{label = "The label", type="bar-style", value = {
"active", --the 1st bar: opacity = 1
"active fgold", --the 2nd bar: opacity = 1 + gold
"active fred", --the 3rd bar: opacity = 1 + red
"possible fred",--the 4th bar: opacity = 0.5 + red
"possible", --the 4th bar: opacity = 0.5
"", --the 5th bar: opacity = 0.2
}},
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Icon
A statistic with icons on the right
Syntax
{label = "", type = "icon", value = {{icon = '', opacity = 1.0}}}
Keys
label
: string
the left label
type
: string
value
: table
A list of table to define the icon from left to right, 1 table = 1 icon
icon
: string - Icon filename fromnui\menu\assets\images\icons\
folderopacity
: float (0.0<>1.0) - The opacity of the icon
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
statistics = {
{label = "The label", type="icon", value = {
{icon = "player_health", opacity = 1}, --the 1st icon
{icon = "player_health", opacity = 0.75}, --the 2nd icon
{icon = "player_health", opacity = 0.3} --the 3rd icon
}},
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Texts
Basic statistic with two labels
Syntax
{label = "", value = ""}
Keys
label
: string
The left label
value
: string
The right label
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
statistics = {
{label = "The label", value = "The value"}
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)
Weapon-bar
A statistic with the weapon bar design. Useful to display a percent of completion
Syntax
{label = "", type="weapon-bar", value = {current,max}}
Keys
label
: string
The left label
type
: string
value
: table
The percent of completion is calculated by
value.current\value.max
value.current
: float - the current value of the statisticvalue.max
: float - the max value the statistic can reach
Example
local menu = jo.menu.create('menu1',{})
menu.addItem({
title = "Item",
statistics = {
{label = "The label", type="weapon-bar", value = {60,100}}
}
})
menu:send()
jo.menu.setCurrentMenu('menu1')
jo.menu.show(true)